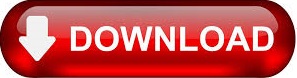
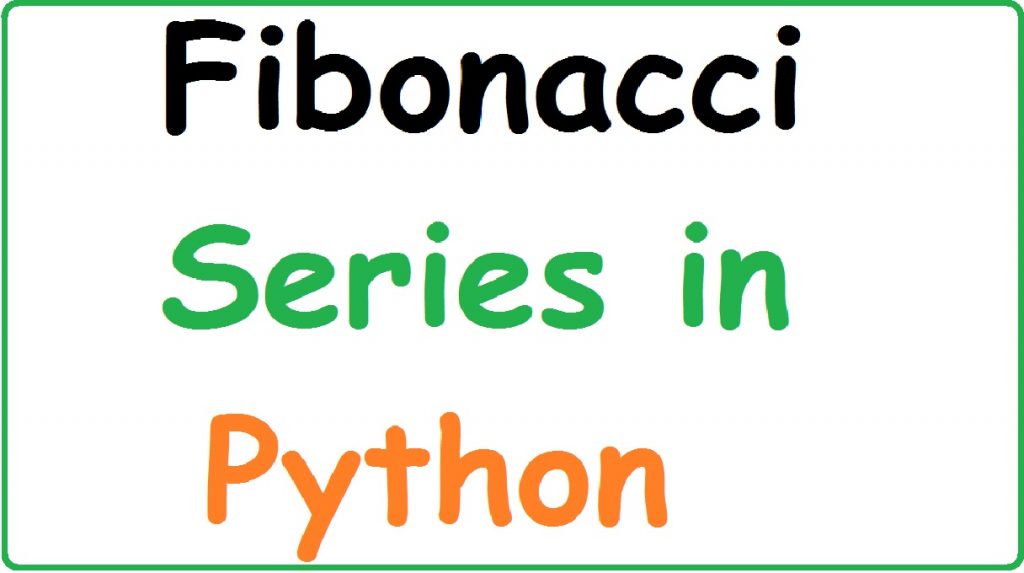
- Fibonacci sequence python how to#
- Fibonacci sequence python generator#
- Fibonacci sequence python code#
You can also use a generator function to make that all easier: def fibGenerator(): That way you don’t have to do the same things over and over again. You should define it outside of it and just reuse it.Īlso, when you know you want to create a list of multiple fibonacci numbers, it helps to just store all the numbers you calculate in between. Then you are redefinining your fib function inside the loop over and over again. Also, you can simplify the whole block by just doing this: numberlist = list(range(20))Īnd given that you don’t actually need that to be a list, you don’t need to construct that at all but you can just run for n in range(20) later. You don’t need to initialize i there, the for loop does that for you. Create a class for both both the squares and cubes which takes parameter n and returns a list of the squares/cubes.Also some more comments on your code: numberlist =
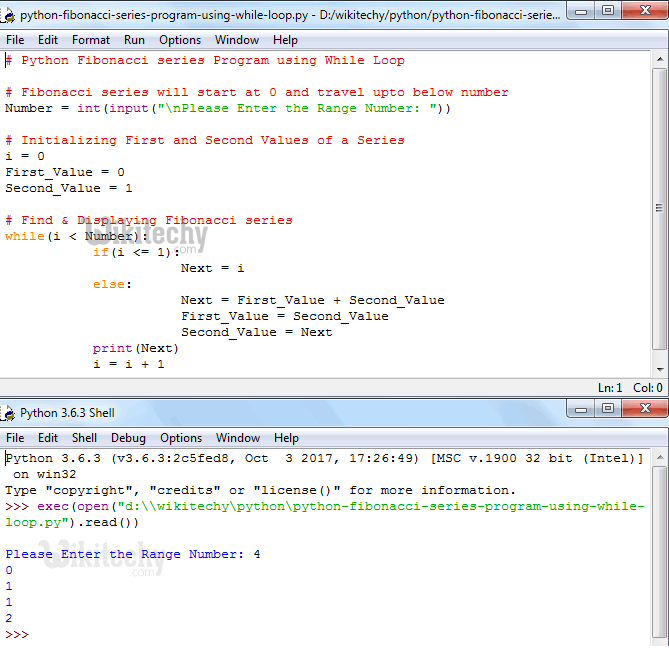
Notice the increase in performance for our final Fibonacci example. We can do a lot of customization to suit the use case by learning about these items. _iter_ and _next_ are known as the iterator protocol when implemented in a class. _getitem_ and _setitem_ are analogous to the getter and setter which were discussed in the properties section . This is a good example of using OOP to customize objects to suit our needs. Since sets are not subscriptable we will still keep the list of Fibonaccis but ensure that when in is called we only use sets for performance reasons. Notice how much faster this is compared to the previous method. Return Fibonacci.fib(n-1) + Fibonacci.fib(n-2)
Fibonacci sequence python how to#
We will use this method to demonstrate how to implement some important methods. This method can be imported from Python's built-in library functools.
Fibonacci sequence python code#
We will use the LRU cache here as it will make the code run faster. Let's take a look at a function from the Python docs for calculating the n th Fibonacci number. Obviously we probably wouldn't want to only have the first 10 Fibonacci numbers in an object. So although this is a very contrived example, hopefully this demonstrates the ability for us to customize the behavior of our code. Raise TypeError("Not allowed to modify the Fibonacci Numbers") But we can implement the method and add a custom error message if someone tries to set values in the way discussed above. In our case we probably don't want to be able to be able to allow setting the Fibonacci numbers, but clearly in many cases we will want it. Recall that with normal lists in Python we can set an element in a list using the following syntax:īehind the scenes Python is using the following: Since we have the _len_ method defined in our class we can also loop through all the elements using a common looping technique.
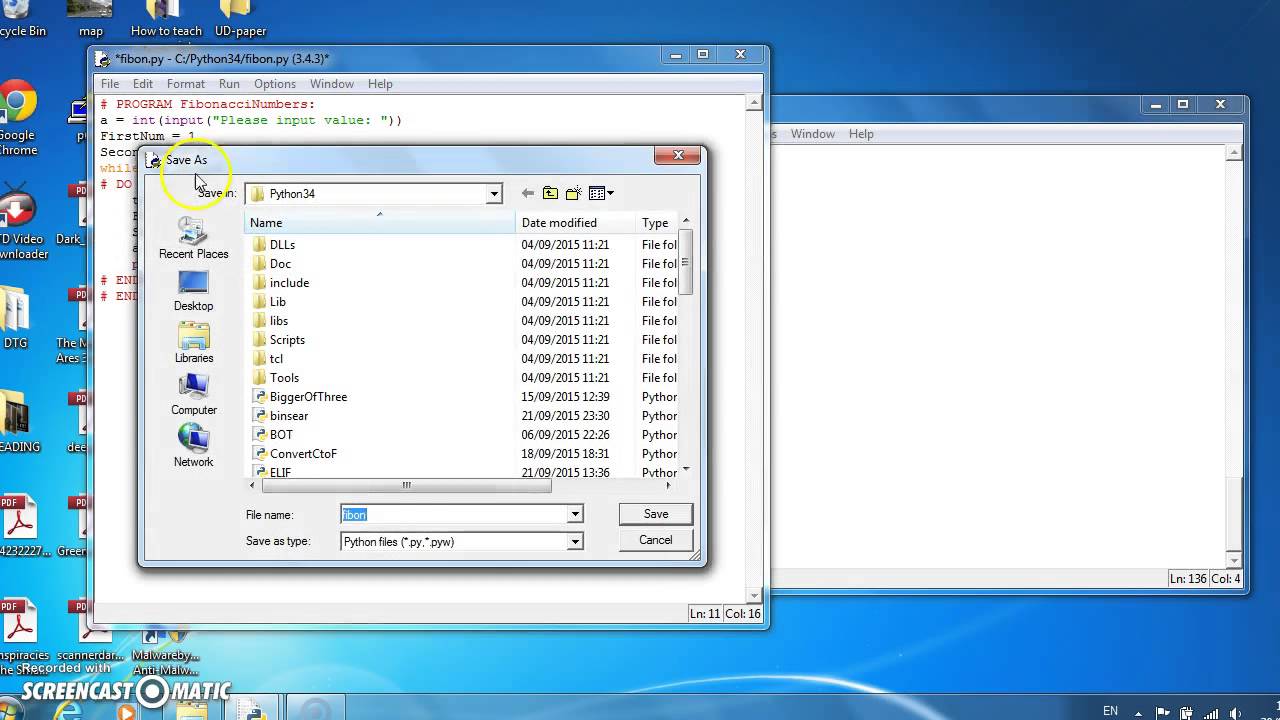
We also implement the _len_ method to allow us to call the len() keyword on an instance of our object. First let's see how this method works with Python's built in list objects. Therefore if we want to be able to access elements in the typical way there are a few methods we will need to implement.īelow we define a _getitem_ method which returns the elements based on the given slice_ we pass through to the method. If you ever see the TypeError: object is not subscriptable exception , the reason why is that a special method has not be included in the class. TypeError: 'Fib10' object is not subscriptable
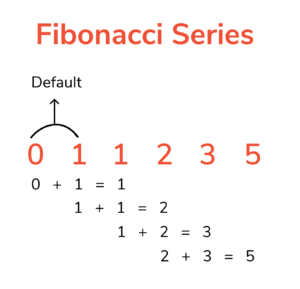
Trying this with an instance of our Fib10 class results in the following: Say we wanted to be able to access the elements within this class the way we typically do with lists in Python. Let's begin with a simple object that contains the first 10 Fibonacci numbers. In this article we will focus on lists and generators, however the same methods can be used for dictionaries.įirst recall how we usually access elements of a list in Python mylist = We will use the Fibonacci sequence to demonstrate these concepts, since they are also quite a popular interview question, hopefully this will make these concepts easy to remember. This article will give an overview on how to use them. Sequences are quite possibly the most widely used data structure in programming. Python Sequence Objects with Fibonacci Example
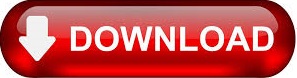